摘要:实现word、excel、ppt的在线预览
onlyoffice官网地址:https://www.onlyoffice.com/
安装onlyoffice
参照官网文档进行安装:
https://helpcenter.onlyoffice.com/server/docker/document/docker-installation.aspx
通过docker方式安装:
1 2 3 4 5
| sudo docker run -i -t -d -p 9002:80 --restart=always \ -v /app/onlyoffice/DocumentServer/logs:/var/log/onlyoffice \ -v /app/onlyoffice/DocumentServer/data:/var/www/onlyoffice/Data \ -v /app/onlyoffice/DocumentServer/lib:/var/lib/onlyoffice \ -v /app/onlyoffice/DocumentServer/db:/var/lib/postgresql onlyoffice/documentserver
|
安装完成后访问 http://localhost:9002/ 即可看到欢迎界面
在项目中使用
1. 引入js文件
1
| <script src="http://localhost:9002/web-apps/apps/api/documents/api.js"></script>
|
2. 参考api编写预览方法
详细配置文档:https://api.onlyoffice.com/editors/config/
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
|
function preview(url, filename) { const index = filename.lastIndexOf('.'); const fileType = filename.substr(index + 1); const config = { "document": { "permissions": { comment: false, fillForms: false, "edit": false, }, "fileType": fileType, "title": filename, "url": url, "lang": "zh-CN" }, "width": '100%', "editorConfig": { mode: 'view', "lang": "zh-CN" } }; document.title = filename; const docEditor = new DocsAPI.DocEditor("placeholder", config); }
|
3. 调用预览方法
1 2 3 4 5 6
| const url = 'http://localhost:8083/filecenter/file/2c948175730446020173058e906b0015';
const filename = '2020年招生计划.docx';
preview(url, filename);
|
4. 效果
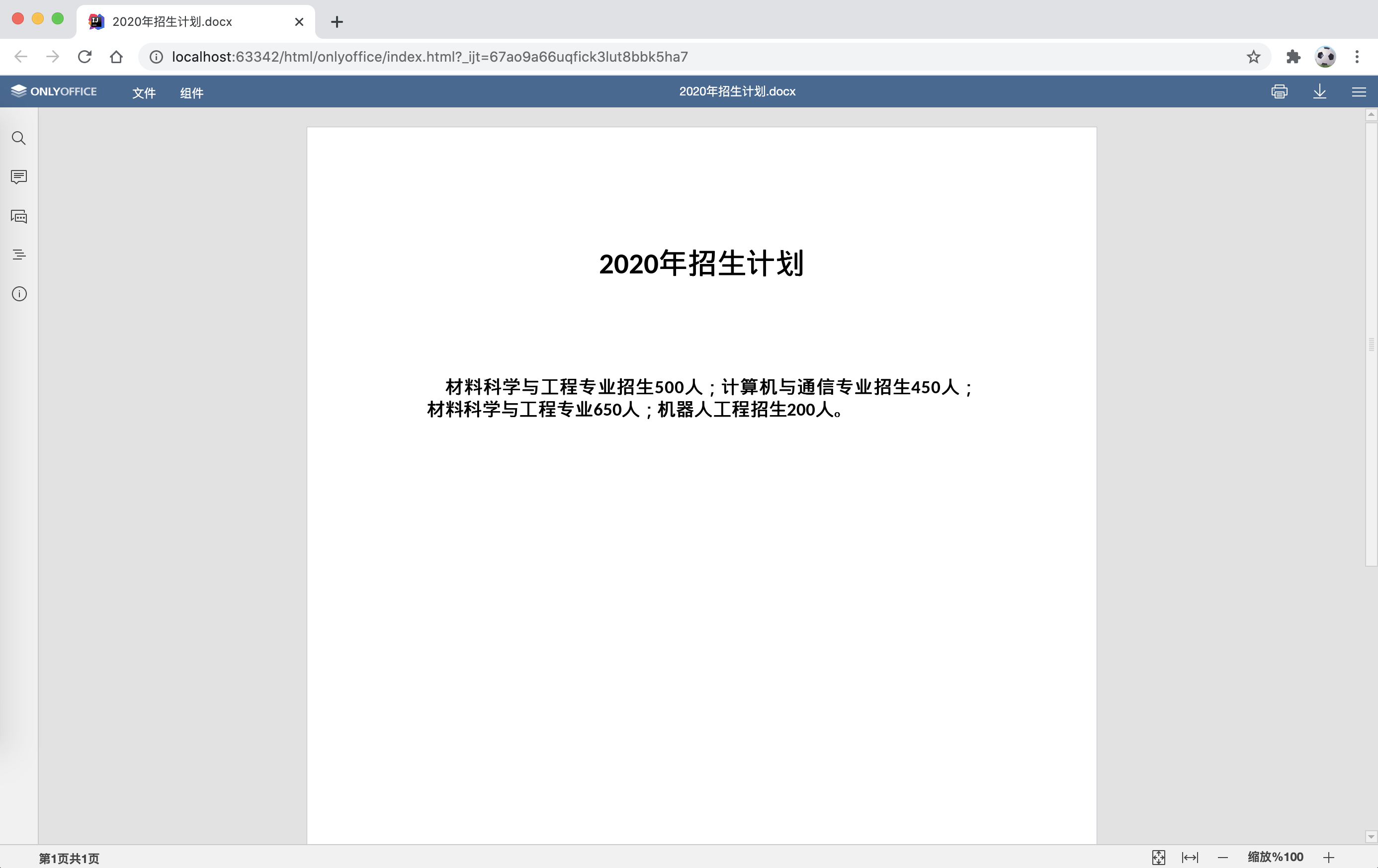
完整代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59
| <html lang="zh-CN"> <head> <title>Demo</title> <meta content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=0" name="viewport"/> <meta charset="utf-8"/> <script src="http://localhost:9002/web-apps/apps/api/documents/api.js"></script> <style> body { padding: 0; margin: 0; } </style> </head> <body>
<div id="placeholder"></div> <script>
function preview(url, filename) { const index = filename.lastIndexOf('.'); const fileType = filename.substr(index + 1); const config = { "document": { "permissions": { comment: false, fillForms: false, "edit": false, }, "fileType": fileType, "title": filename, "url": url, "lang": "zh-CN" }, "width": '100%', "editorConfig": { mode: 'view', "lang": "zh-CN" } }; document.title = filename; const docEditor = new DocsAPI.DocEditor("placeholder", config); }
const url = 'http://localhost:8083/filecenter/file/2c948175730446020173058e906b0015'; const filename = '2020年招生计划.docx'; preview(url, filename); </script> </body> </html>
|